5 Types of Data Structures and Algorithms Computer Scientists Must Know
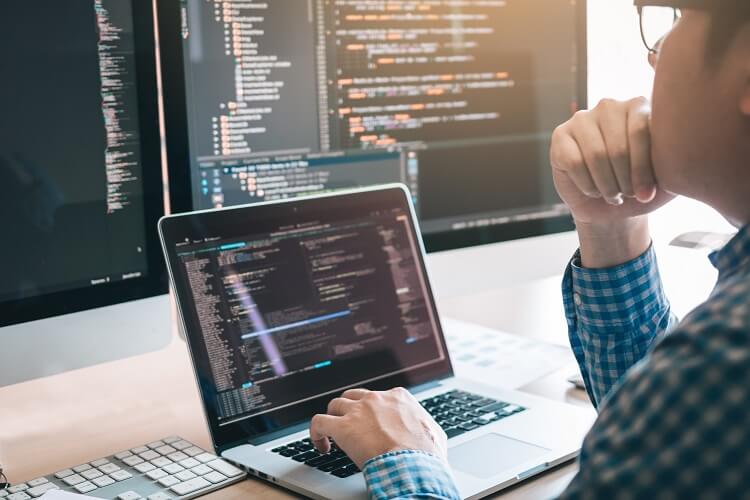
Do you want to build advanced computing expertise? You will need to understand the fundamental data structures and algorithms of computer science.
Data structures and algorithms are essential in all areas of computing, from operating systems and networking to programming languages. Use this blog to explore five types used by today’s computer science professionals.
1. Linear Data Structures
There are two types of computer science data structures: linear and nonlinear. Linear data structures are the simplest, arranging data in a single level. Each element directly links to its previous and subsequent elements. Imagine a stack of playing cards or a shelf of books.
Commonly used linear data structures are arrays, linked lists, stacks, and queues.
Array
Arrays store similar data elements at contiguous memory locations, making it easy to process, sort, and search. As one of the oldest computer science data structures, arrays exist in almost every computing program.
Linked List
A linked list is a series of data elements where each element points to the next. This arrangement lets users effectively insert or remove elements from any position of the sequence.
Stack
A stack is a collection of data elements that follows the “last-in, first-out” (LIFO) rule. A new element added to a stack sits on top of all previously added elements. Only the element on top of the stack can be removed. Stacks are ideal for retrieving recently used objects or data in the order it was entered.
Queue
Queues are collections of data elements that follow the “first-in, first-out” (FIFO) rule. Unlike stacks, queues place a new element at the bottom of all previously added elements. The first element of the queue is always removed first.
2. Nonlinear Data Structures
Graph data structures and algorithms are key computer science concepts. Graphs are nonlinear data structures. They contain multiple levels of data and do not connect elements sequentially. As a result, graphs enable computing professionals to solve complex problems using data.
Graphs consist of nodes and edges. Nodes store data elements, and edges represent the relationships among them. Graphs can show various data relationships, from electrical circuits to the individuals in a social network.
One type of graph is a tree, which arranges nodes hierarchically. The topmost node is the root. Connected to the root are zero or more subtrees. For example, a binary search tree has two subtrees connected to the root, a left subtree and a right subtree.
Because trees are hierarchical, they often represent data such as file systems and directories.
3. Sorting Algorithms
Sorting algorithms are step-by-step procedures for rearranging data in arrays and lists. For example, a user may need to sort an array in numerical or lexicographical order. Sorting algorithms enable other algorithms to run more efficiently, such as searching algorithms.
Insertion sort, merge sort, and quick sort are three fundamental algorithms for sorting.
Insertion Sort
For small data sets that are almost sorted, insertion sort is an efficient way to finish the job. This algorithm divides an array into sorted and unsorted parts. Then it selects an element from the unsorted part and places it in the sorted part until all of the elements are sorted.
Merge Sort
Merge sort is ideal for organizing linked lists. It divides a list into two halves until it cannot be further divided. Then it compares and merges elements back together in the same way they were divided.
QuickSort
Large data sets benefit from quicksort. It partitions an array in two subarrays based on a specified data element, called the pivot. The first subarray holds elements with values less than the pivot. The second subarray has elements with values greater than the pivot. The algorithm finds the pivot in each subarray until all subarrays contain only one element.
4. Searching Algorithms
Searching algorithms find and retrieve specific elements from data structures. Two key examples are linear search and binary search.
Linear Search
Linear search is a sequential searching algorithm for sorted and unsorted data structures. It traverses lists and arrays sequentially, one element at a time.
Imagine an unsorted list of elements with values “1” through “25.” A linear search for the value “5” would traverse the values in the order they are stored until it’s located.
Binary Search
Binary search is an interval searching algorithm . Interval searches divide elements into multiple intervals and only traverse the expected interval. They are more efficient than sequential searches because they divide the search space.
Binary search is efficient for finding elements of a sorted list. It compares the search value to the middle element of the data structure and then traverses the expected interval.
Consider a sorted list of elements with values “1” through “25.” A binary search for the value “5” would compare it to the middle element, which is “13.” Because “5” is less than “13,” the algorithm would search the lower half of the interval for the value.
5. Graph Traversal Algorithms
Computer scientists use graph traversal algorithms to search nodes in graphs and trees. Unlike linear computer science data structures, graphs must be searched more than once to find and retrieve data.
Two algorithms for traversing graphs are breadth-first search and depth-first search. They help computing professionals solve the most common problems involving graphs and trees.
Breadth-First Search
Breadth-first search (BFS) traverses the shortest path between two nodes. Starting at the tree root, it explores nodes in order of distance.
In a tree with two levels of nodes, BFS would search nodes from left to right in the following order:
-
Tree root
-
Nodes in the first level
-
Nodes in the second level
Depth-First Search
Depth-first search (DFS) searches graphs from top to bottom . It traverses as far as possible down a single branch before backtracking to continue traversing the next.
There are three ways to apply DFS:
-
Preorder Traversal: Starts at the tree root, then traverses the left subtree, followed by the right subtree.
-
In-order Traversal: Starts at the left subtree, then traverses the tree root, followed by the right subtree.
-
Post-order Traversal: Starts at the left subtree, then traverses the right subtree, followed by the tree root.
Build Advanced Computing Skills to Advance Your Technology Career
Whether you’re looking to switch careers or go further into the realm of computing, developing your computer science knowledge will prepare you for the fastest-growing career paths. Understanding the data structures and algorithms of computer science is just the beginning.
Worcester Polytechnic Institute (WPI) offers a Master of Computer Science Online program that will help you chart your own course for a dynamic future in computing. As a student, you will establish a foundation in software, algorithms, and data management and personalize your degree path.
Graduates of the Master of Computer Science Online program have mastery of the following:
-
Foundations of Computing: Gain essential knowledge of programming concepts, systems, networking, and data structures and algorithms in computer science.
-
Computing Design: Understand the design and analysis of software systems, algorithms, and database management systems.
-
Specialized Computing: Tailor your degree to your career aspirations by choosing from concentrations in Computing Systems or Cybersecurity—or build your own area of focus from a range of electives.
WPI’s educational excellence has received recognition from organizations and prestigious publications across the country, including:
-
Top 50 Most Innovative Schools (U.S. News & World Report)
-
Top 25 STEM Colleges (Forbes)
-
#20 Best Value College/Top Return on Investment (Payscale.com)
Are you ready to grow your career in technology? WPI can help.
Learn more about the Master of Computer Science program at WPI.